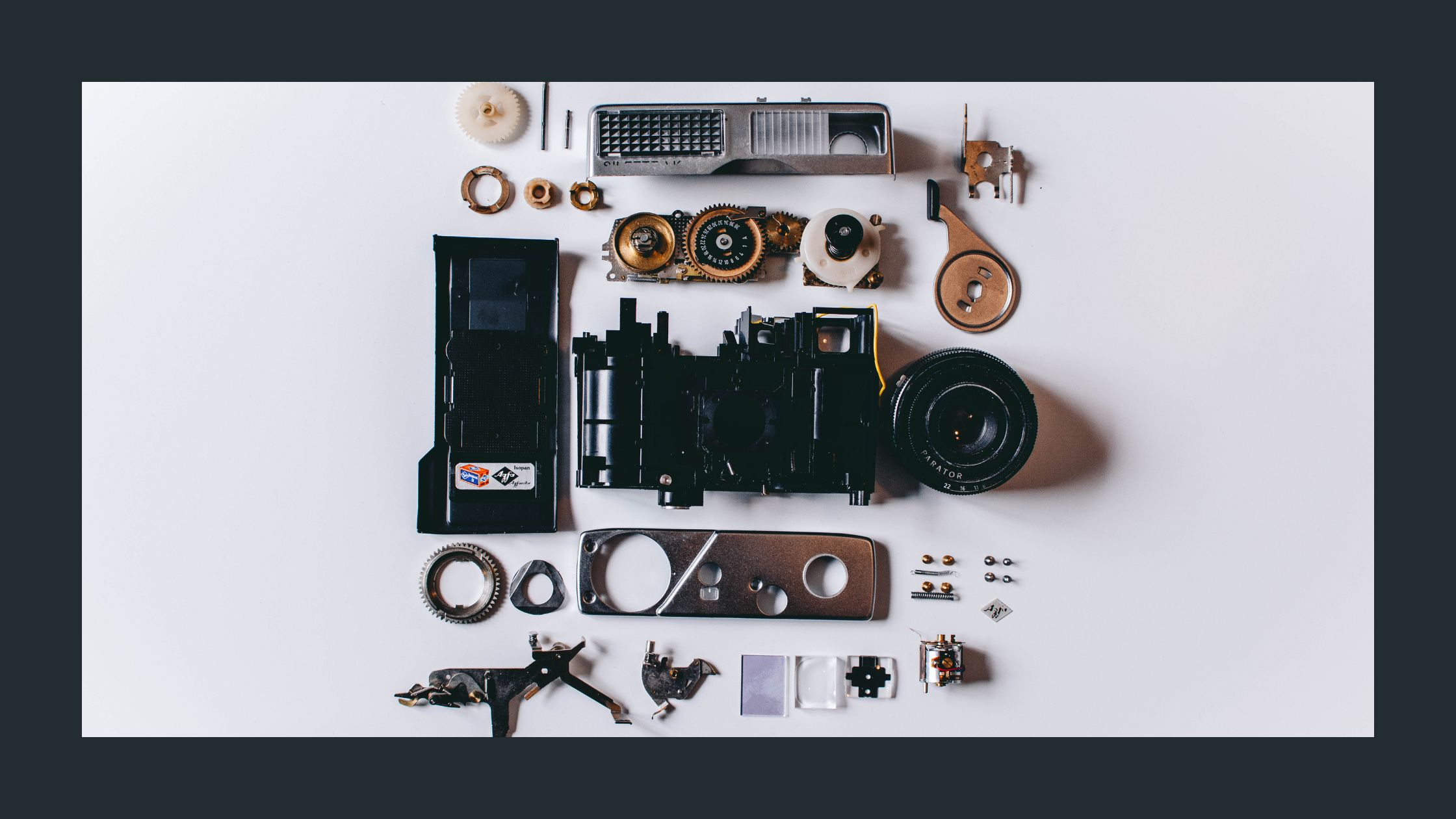
Programmers are writers for computers using a programming language. They write lists of instructions. Computers read and follow the lists but they do not rationalize the given instructions. Computers just follow instructions without question.
A computer understands Binary Code which consists of only 0’s and 1’s. That is the only thing that the computer reads. So writing instructions in binary code can get very complicated. That is why programming languages were created.
An example of binary code would be the following representation of the letter a, in lower case:
a = 01100001
Notice how many 0’s and 1’s are required for the computer to read a representation of the letter “a”. In case you were wondering, there are eight digits ( five 0’s and three 1’s) total to represent one character (“a”).
As a programmer, you do not need to understand how keyboard characters relate to 0’s, and 1’s when using most of the current programming languages. Furthermore, you do not need to understand how they convert or compile, from programming language to binary code. You just need to understand the preferred programming language, which is easier to follow than a bunch of 0’s and 1’s.
Using the English language as a reference to understand parts of the programming language
There are different approaches to learning a new language. Some people decide to learn a language that has the same root as their native language. This approach may help to learn faster because of how easier it may be to assimilate words and concepts between languages. For example, if a native English speaker begins to learn German, words like Willkommen will resonate fast because it sounds similar to, and means the same as Welcome.
Most of the programming languages use keywords from English. So it helps if you know the language. But even though it uses English, programming can be too technical and too abstract to understand when reading at first.
How can it be easier to assimilate words and concepts of the programming language with a native language, this case English language?
Every language has its structure. So, let’s extrapolate the general idea of the different structural parts of English. Forget for a moment about the technical aspect of programming. Focus on the general parts that compose the language. Let’s compare general parts of the English language with general parts of most programming languages.
Data of the Function as the Subject of a Sentence
A known concept from a programming language is Data. We hear about data everywhere. Data is information that you want to manipulate, store, or delete. An example of data is the following sentence:
'My name is Yari Antonieta'
Also, programmers use variables to store data that affect how the program works. An example of a variable storing data is:
let example = 'My name is Yari Antonieta';
The example above shows “example” as the variable storing the data “‘My name is Yari Antonieta’ “.
When writing in English, the subject of a sentence is a person, place, thing, or idea that is doing or being something.
Do you see where I am going here?
I am generalizing the idea of the main thing of which the writer is referring to. In English, it is known as the subject in a sentence. While in a programming language can be seen as data of the function.
Data Types as Adjectives of the Subject
Descriptions provide more information about the object that is being referred to. Adjectives are words that describe the subject of a sentence in the English language. Words like several, tall, and blue are examples of adjectives commonly used.
In a programming language, Data is defined or described by its Type. Data Types describe what type of information the data is. There are multiple types of data because there are multiple types of information formatting.
Data Type Numeric is information provided is composed of numbers. True or false values are Data Type Boolean. Other types of data include arrays, objects, characters, dates, long, integers, and so on. Some languages let you define your data type.
Let’s do a quick exercise! Can you answer which data type is the variable “example“? See below. I will be providing the answer at the end of this post.
let example = 'My name is Yari Antonieta';
Data Structure and Sentence Structure
English and also programming languages depend on the structure of their components. It is so that the message or instructions provided can be easily read by the receiver end.
As discussed earlier, the Data Types describe the type of information that we are dealing with. If a set of data is declared or defined as an array, the data must, at a minimum, be inside a square bracket ( [ ] ).
The Data Structure is the format in which the information has to be stored to comply with the variable declaration. An example of an array variable declaration:
let box = ['a', 'b', 'c'];
Assimilating the English language, the structure of a question has to have at least a question mark (?). A question may also have an interrogative adjective or verb (Who, What, When). Another example could be a simple sentence in which you need a subject, predicate and a punctuation mark (.).
Another quick exercise! Can you answer which data structure the variable “example” has? See below. I will be providing the answer at the end of this post.
let example = 'My name is Yari Antonieta';
Regular expressions as math equations
If languages have mathematical equations, then programming languages have regular expressions. Regular Expressions are like a search engine for a given set of characters. It searches for string patterns.
An example of why you may need a Regular Expression is when validating an email address on a submitted form. The usual format of an email address has an at (@) and a .com, .org, or .something at the end. From https://www.regextester.com/19 and email Regular Expression looks like this:
const email = ^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$
Yikes! That looks so complicated. And well, for me it still is. With that in mind, I will not dive into this subject, for now.
Logical Operators and Conditional Expressions as Questions
Computers are programmed to make decisions even though it does not rationalize what it reads. Programming languages provide logical operators and conditional expressions to instruct computers on what conditions are needed to perform a task.
A simple way to instruct the computer to return true if the value is equal to three and false if the value is not equal to three would be:
let i = 3;
if (i === 3) {
return true;
} else if (i !== 3) {
return false;
}
Be aware because logical operators and conditional expressions may seem easy to handle but they are tricky. So tricky in fact that they can cause apps or programs to crash. They even can make a computer loop a program indefinitely!
Functions and Methods working as Paragraphs
A paragraph’s structure has a body that consists of various sentences, or questions. These parts are arranged, within certain rules, to deliver a complete descriptive message.
A Function is a small list of instructions within the larger list of instructions. It’s a way of organizing the tasks the computer should perform.
Like a paragraph’s structure, the function has a body where various parts of the programming languages such as a variable declaration, or regular expression verifications are included.
Methods are manipulations performed to the general data to extract the desired data. Also considered as a smaller list of instructions within the function.
The composition of multiple paragraphs results in an essay, article, blog post, while the composition of multiple functions and methods results in Software.
Learn computer language
This is a personal interpretation of how I assimilate these general concepts or ideas of parts of the programming language with the English language.
Programming can be challenging. Hopefully, this exercise helps in providing at least a high-level understanding of the before-mentioned concepts to move forward with your self-taught programming, or coding journey.
If you have never taken a computer science, web development, or algorithm course, it is required for you to take time to see, read, or hear what a program is, and how does it work.
Learning to Program from Steven Foote (Senior Software Engineer at LinkedIn when this was published) is an excellent book to break the programming ice. It explains everything from what a program is, how does it work, the various programming languages, and their differences.
Check out this post I wrote: “Resources for Newbie Coders” if you need more resources to learn to program. I update the post every time I find a helpful source.
Just dedicate time and practice for you to get better at programming. With time, you will be able to recognize patterns. Furthermore, programming languages are similar between themselves, so the more programming languages you learn, the easier it will get to learn new languages to come.
Answer for Data Types as Adjectives of the Subject Section Question: the variable “example” is a string Data Type.
Answer for Data Structure and Sentence Structure Section Question: the variable “example” is a string Data Type that has a Data Structure of characters between quotation marks (‘ ‘).
Thanks for reading.
You can get a new post notification directly to your email by signing up at the following link.
Related Articles
The following CTRL-Y articles are related somewhat to this post. You may want to check them out!:
- My First Year Writing Code
- Resources for Newbie Web Coders
- 5 Reasons Why Women Should Consider a Career in Web Development
- A Morning in a Front-End Developer’s Mac Terminal
- Learn to Code
- Incorporating Digital Assets into Your Finances
- Filtering with GraphQL and Prisma: What NOT to Do
By the way – A playlist for you
I wrote this post while listening to This is Mozart playlist on Spotify. My head wanted to hear some classical music while writing. I had to work a lot in front of the computer that day. I was very productive while hearing this playlist because it gave me a lot of stimuli.
Can you assimilate any human language’s structure with a programming language? Am I crazy to think about that comparison? Comment below!